It was the start of 2021, lockdown was still in full swing and i was bored…
So what do you do? Gyms were closed, Bouldering was on hiatus, Restaurants were out of service… You could only go to the Supermarket, work, Amazon and the 2dollar store… So… you make robot teeth off course?!?!?!
The idea was a bit simple, get a little microcontroller, learn how to program, buy some of those wind up teeth, and figure out a way to combine everything so you can remote control the teeth to move.
The Teddy’s (equivalent of a 2 dollar store) had these funny looking teeth with eyeballs, and i just bought an ESP32 Dev board and finally i got the cheapest servo i could find.
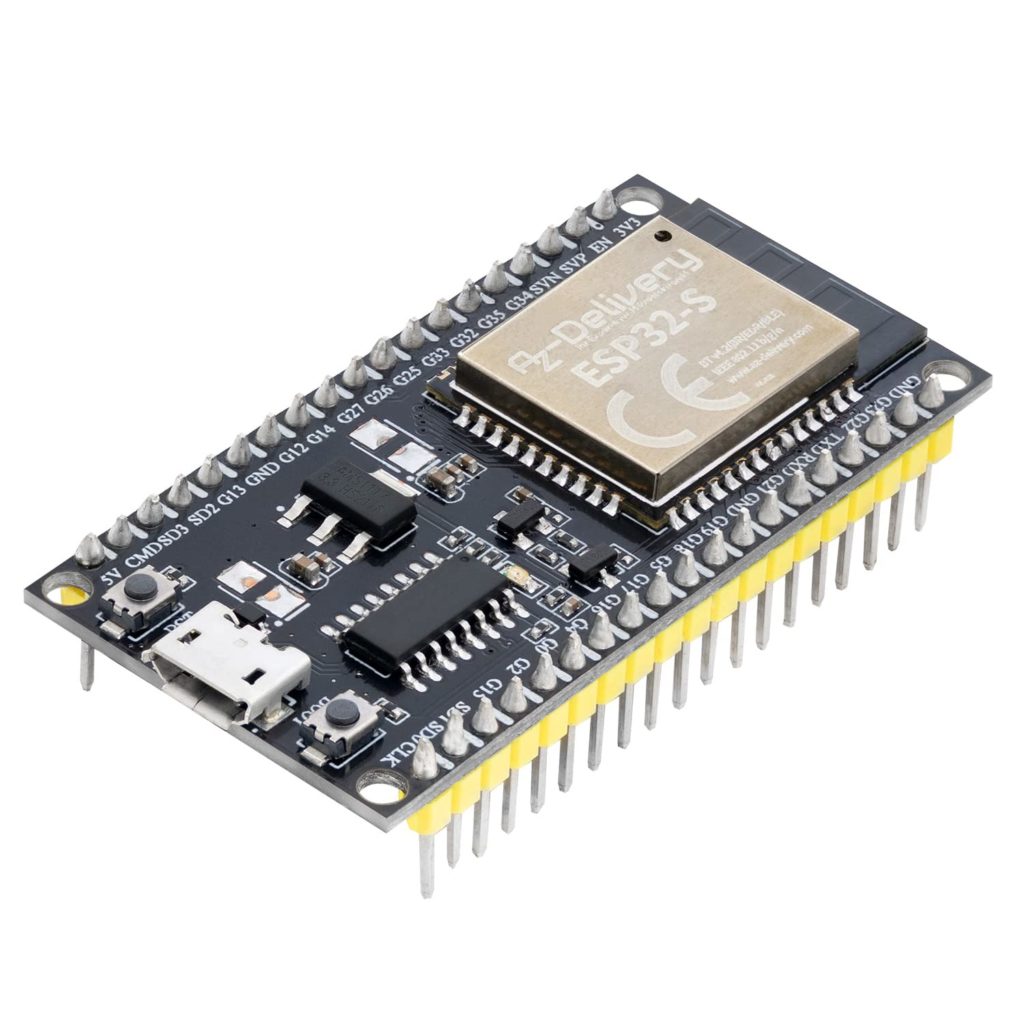
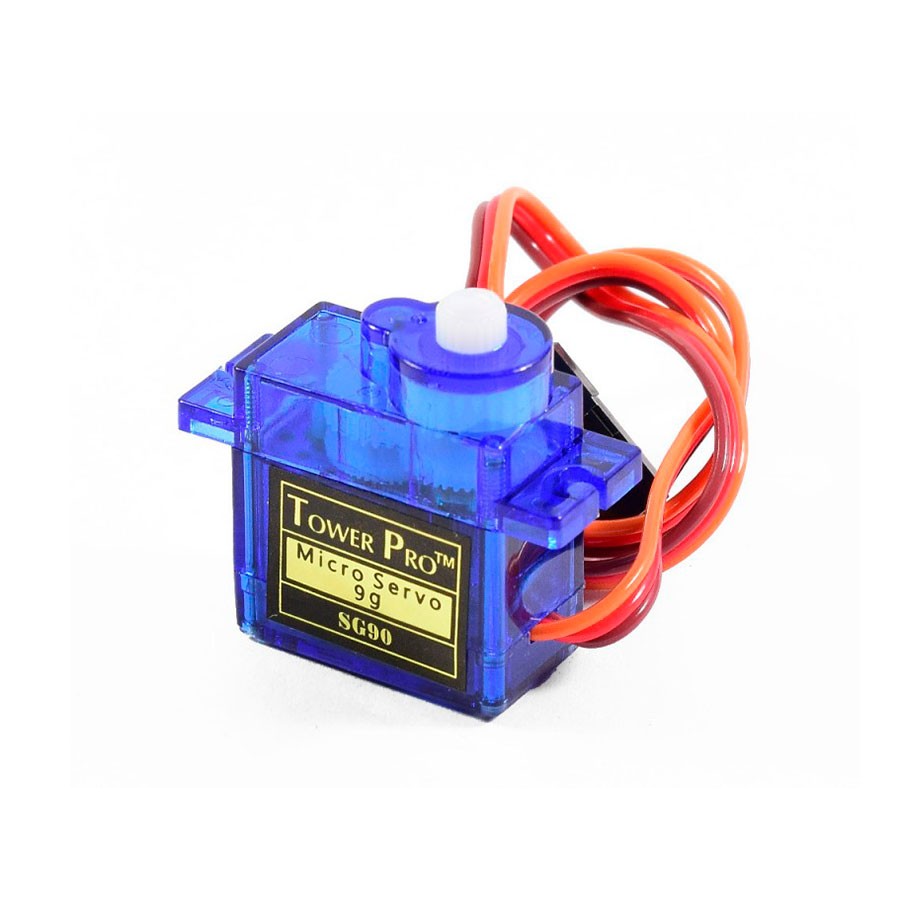
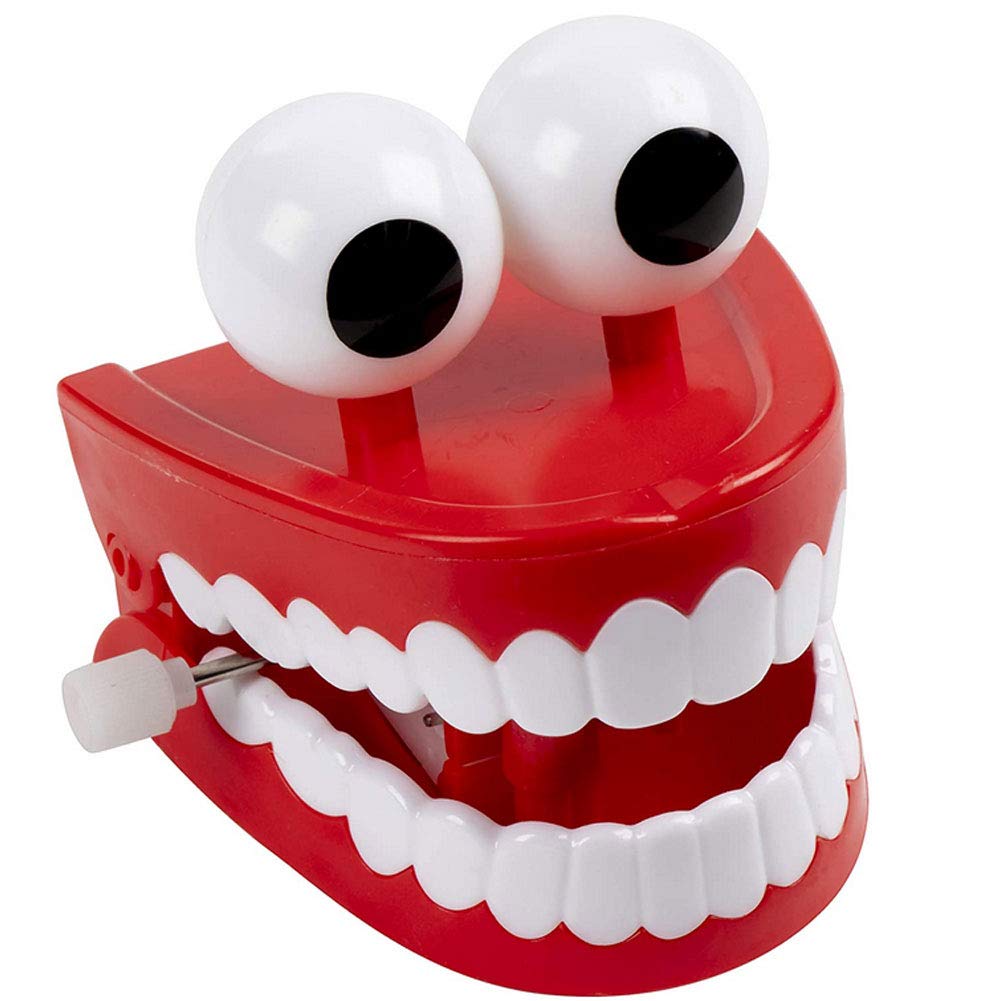
I had to remove the winding box from the teeth with a little bit of brute force. The mechanism is pretty simple, the winding box had a triangle that turns. The top part has a small protruding piece of plastic, and each time the triangle has one of the edges pointing up it pushes up the plastic of the top part, and each time the flat side of the triangle is up, the top part rests on it in a down position. By removing the winding box, and replacing it with the servo, i could now write a tiny script to control the up and down movement myself.
The setup was as followed, there was a 1K resistor between the button input and the ground. Power was connected to the 3v3 pin (red), ground (black) was connected to the left GND pin, pin 35 (yellow) of the ESP32 was used to listen for the button, pin 13 (blue) was used to control the servo inside the teeth. And the servo was put inside the teeth with a single
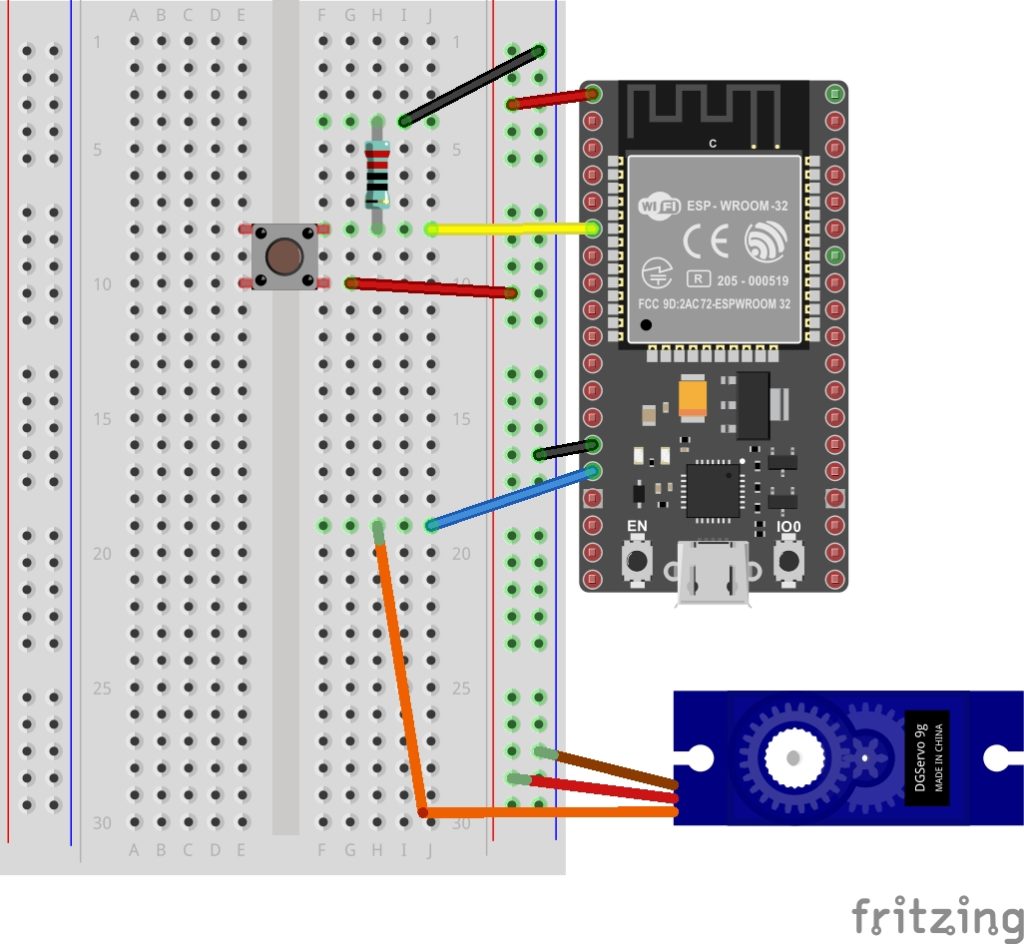
Then i used the Servo.h library to control the servo, and used the following Arduino code to control the teeth with the button.
#include <Servo.h>
Servo myservo_mouth; // create servo object to control a servo
Servo myservo_eyes_x; // create servo object to control a servo
Servo myservo_eyes_y; // create servo object to control a servo
// twelve servo objects can be created on most boards
int pos_mouth = 0; // variable to store the servo position for mouth
const int buttonPin = 35; // the number of the pushbutton pin
int buttonState = 0;
void setup() {
myservo_mouth.attach(13); // attaches the servo on pin 13 to the servo object
myservo_mouth.write(pos_mouth);
pinMode(buttonPin, INPUT_PULLUP);
Serial.begin(115200);
}
void loop() {
buttonState = digitalRead(buttonPin);
if (buttonState == HIGH) {
for (pos_mouth = pos_mouth; pos_mouth <= 160; pos_mouth += 1) { // goes from 0 degrees to 160 degrees
myservo_mouth.write(pos_mouth); // tell servo to go to position in variable 'pos'
delay(2);
}
}
if (buttonState == LOW) {
for (pos_mouth = pos_mouth; pos_mouth >= 0; pos_mouth -= 1) { // goes from 160 degrees to 0 degrees
myservo_mouth.write(pos_mouth); // tell servo to go to position in variable 'pos'
}
}
}
The code makes it so that if you push the button the teeth go up (semi-slowly) and when you release the button, it closes. It’s a bit jittery because i don’t do any sort of smoothing here, and the CPU is probably much faster than the servo can keep up with so it probably skips certain steps, but this is only V1. The end result looked as followed.